What is the MoveVM?
WTF Is MoveVM?
MoveVM is a blockchain virtual machine designed to execute smart contracts written in the Move programming language. Initially developed by Meta (formerly Facebook) for its Libra (Diem) blockchain, MoveVM has since been adopted by projects like Aptos and Sui. It is optimized for security, efficiency, and formal verification, making it a strong alternative to traditional virtual machines like Ethereum’s EVM and Solana’s SVM.
1. History of MoveVM
MoveVM was introduced as part of Meta’s Libra blockchain project in 2019. Libra aimed to create a global payments system, but regulatory challenges led to its rebranding as Diem. Even after Meta abandoned the project, Move and MoveVM continued evolving independently. Now, projects like Aptos and Sui leverage MoveVM for their smart contract execution due to its security-focused architecture.
2. Move Overview
Move is a Rust-based smart contract programming language designed with security and asset safety in mind. Unlike Solidity (EVM) or Rust (SVM), Move treats digital assets as first-class resources, preventing issues like double-spending or accidental loss.
2.1 Peer-to-Peer Payment Transaction Script
Move’s architecture ensures that only authorized users can transfer assets, preventing unintended token duplication or loss. Below is a breakdown of key elements in a Move transaction:
- 0x0 → The account address storing the module.
- Currency → The module name.
- Coin → A resource type representing a digital asset.
- move() → Transfers a resource, making it unavailable for reuse.
- copy() → Duplicates a value, but not applicable for resources.
Code Breakdown
- The sender calls
withdraw_from_sender
from the0x0.Currency
module. - The sender moves the
Coin
resource to the payee viadeposit
.
Rejected Code Examples
- Duplicating Currency (
copy(coin)
) → Results in a verification error as Move enforces resource ownership. - Reusing Currency (
move(coin)
twice) → Would allow double-spending, which is prevented by Move. - Losing Currency (Neglecting
move(coin)
) → Causes a verification error to prevent asset loss.
2.2 Currency Module Features
deposit()
→ Can be called by anyone.withdraw_from_sender()
→ Restricted to the coin owner.GetTxnSenderAddress
→ Similar to Solidity’s msg.sender.RejectUnless
→ Equivalent to Solidity’srequire
, ensuring transaction validity.Pack<T>
→ Creates a new resource type.Unpack<T>
→ Can only be called inside the declaring module.
3. Why MoveVM Is Better Than EVM & SVM
3.1 Security & Formal Verification
- MoveVM ensures safe resource management with built-in ownership rules, preventing token duplication, re-entrancy attacks, and unintended asset loss.
- Formal verification allows developers to mathematically prove the correctness of their contracts, reducing vulnerabilities.
3.2 Performance & Efficiency
- Parallel execution: Unlike EVM, MoveVM supports parallel transaction execution, leading to faster processing times.
- Lower gas costs: More optimized transaction execution than EVM and SVM.
3.3 Storage Model
- MoveVM’s Global Storage Model is more efficient than EVM’s account-based model.
- Ensures strict resource constraints, making it ideal for complex applications.
4. Move Virtual Machine Principle
Move-VM and EVM share a similar process: compiling smart contract code into bytecode and executing it in the virtual machine. However, MoveVM introduces bytecode verification to ensure security before execution.
Execution Flow:
- Load bytecode through
execute_script()
. - Deserialize and verify the script via
load_script()
. If verification fails, execution is aborted. - If valid, execute the bytecode, modifying global storage (resources and modules).
5. MoveVM Technical Architecture
5.1 Global Storage Model

MoveVM’s global storage is structured as a tree, where:
- Each account address stores resources and modules.
- Each resource type has only one instance per address, preventing unauthorized duplication.
Pseudo-code Representation
struct GlobalStorage {
resources: Map<(address, ResourceType), ResourceValue>
modules: Map<(address, ModuleName), ModuleBytecode>
}
5.2 Move Prover (MVP) - Making Smart Contracts Bulletproof
The Move Prover (MVP) is a formal verification tool that makes sure smart contracts are solid, bug-free, and reliable. It works like a compiler or a linter—fast, predictable, and built to be part of the development workflow. Unlike traditional software, smart contracts are way easier to verify because:
- They’re compact in size.
- They run in a controlled, isolated environment.
- Their execution is mostly sequential and deterministic.
Since smart contracts handle serious money and regulatory risks, formal verification is a big deal—nobody wants a billion-dollar bug.
How MVP Became Fast & Reliable
Originally, MVP was slow and unpredictable—it sometimes randomly timed out on complex code. Now, it completes verification in under 30 seconds. The major upgrades that made this possible include:
- Alias-Free Memory Model – Move's memory system works like Rust, meaning no accidental aliasing, which makes verification easier.
- Fine-Grained Invariant Checking – Invariants are checked at every state, unless explicitly suspended.
- Monomorphization – Generic types and functions are pre-instantiated, making verification way faster.
These optimizations transformed MVP into a tool that’s not just fast but also reliable—it doesn’t randomly break or timeout anymore.
How MVP Works
MVP runs like a linter—fully automated, quick, and built for dev workflows. Take the example of a Move contract handling account balances:
module Account {
struct Account has key { balance: u64 }
fun withdraw(account: address, amount: u64) acquires Account {
let balance = &mut borrow_global_mut<Account>(account).balance;
assert(*balance >= amount, Errors::limit_exceeded());
*balance = *balance - amount;
}
fun deposit(account: address, amount: u64) acquires Account {
let balance = &mut borrow_global_mut<Account>(account).balance;
assert(*balance <= Limits::max_u64() - amount, Errors::limit_exceeded());
*balance = *balance + amount;
}
public(script) fun transfer(from: &signer, to: address, amount: u64)
acquires Account {
assert(Signer::address_of(from) != to, Errors::invalid_argument());
withdraw(Signer::address_of(from), amount);
deposit(to, amount);
}
}
When you run MVP on this, it catches errors automatically—like missing abort conditions. For example, if withdraw
doesn’t specify what happens when there’s not enough balance, MVP throws an error and provides a counterexample showing how it failed.
Move Specifications & Proofs
Move has a contract-based spec system, letting devs define conditions on their code:
spec transfer {
let from_addr = Signer::address_of(from);
aborts_if from_addr == to;
aborts_if bal(from_addr) < amount;
aborts_if bal(to) + amount > Limits::max_u64();
ensures bal(from_addr) == old(bal(from_addr)) - amount;
ensures bal(to) == old(bal(to)) + amount;
}
spec fun bal(acc: address): u64 {
global<Account>(acc).balance
}
invariant forall acc: address where exists<Account>(acc):
bal(acc) >= AccountLimits::min_balance();
invariant update forall acc: address where exists<Account>(acc):
old(bal(acc)) - bal(acc) <= AccountLimits::max_decrease();
This ensures that:
- A transfer fails if the sender doesn’t have enough balance.
- A transfer doesn’t exceed account limits.
- Account balances always stay within safe limits.
MVP runs these checks instantly—like a type checker—so you catch bugs before they cause disasters.
MVP’s Architecture
MVP takes Move code + specs as input and runs it through a verification pipeline. The pipeline translates Move’s rules into logical constraints, which are fed into an SMT solver (a fancy theorem prover). If something doesn’t check out, MVP provides human-readable errors, making debugging straightforward.
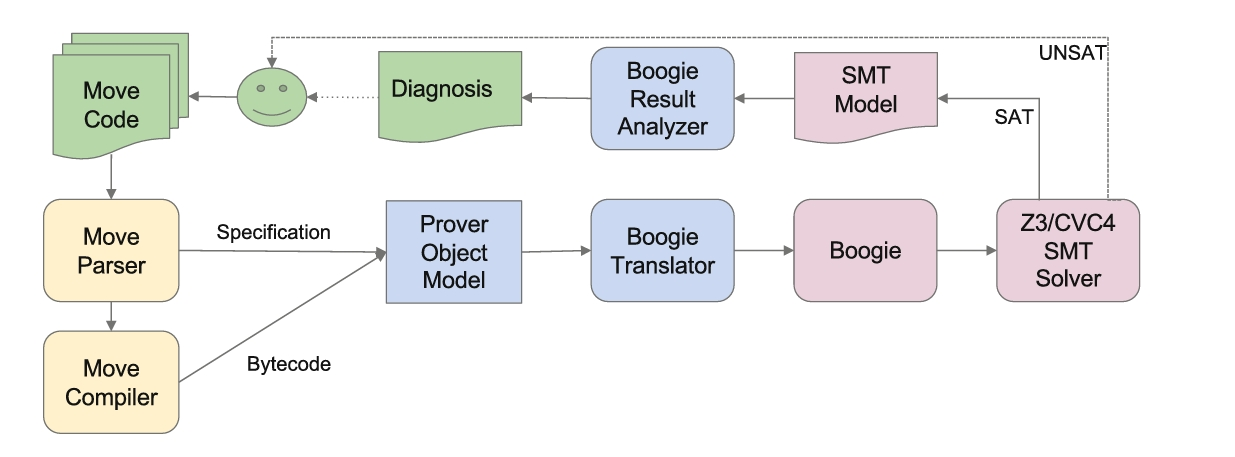
6. Challenges & Future Direction
6.1 Future of the MoveVM Ecosystem
MoveVM is gearing up to be a big player in the smart contract space. Originally built for Diem, it's now powering ecosystems like Movement Labs , Aptos & SUI, with more chains exploring its potential. Its safety-first design, formal verification, and resource-based model make it a top pick for devs who want security without giving up performance. As modular blockchains and parallel execution gain traction, MoveVM could become the go-to runtime for next-gen Web3 apps. Expect cross-chain integrations, zk-powered verifications, and L2 expansions to push Move further into the mainstream.
Nexio
Nexio brings MoveVM to Bitcoin, enabling secure, high-performance smart contracts directly on the world's most decentralized blockchain. Unlike EVM-based Bitcoin Layer 2 solutions, Nexio leverages Move’s built-in security features and precise resource management—delivering improved safety, speed, and efficiency. This opens the door for Bitcoin-native DeFi, NFTs, and essential infrastructure. By combining Bitcoin’s robust stability with Move’s flexible programmability, Nexio is expanding Bitcoin’s capabilities, creating a powerful execution environment that can significantly advance Bitcoin’s smart contract ecosystem.